Since .NET has gone to RC1, I’ve been playing around with the new framework. As a result, I’ve been running into lots of bugs and ‘features’, with the new codebase.
For instance, here’s an interesting bug related to the Entry control.
To duplicate, start by creating a new .NET MAUI project, in Visual Studio 2022.
I chose to use the .NET MAUI project templates from Vijay Anand EG, available from HERE, or from the Visual Studio marketplace. Any .NET MAUI project template will do though, you don’t have to use this one.
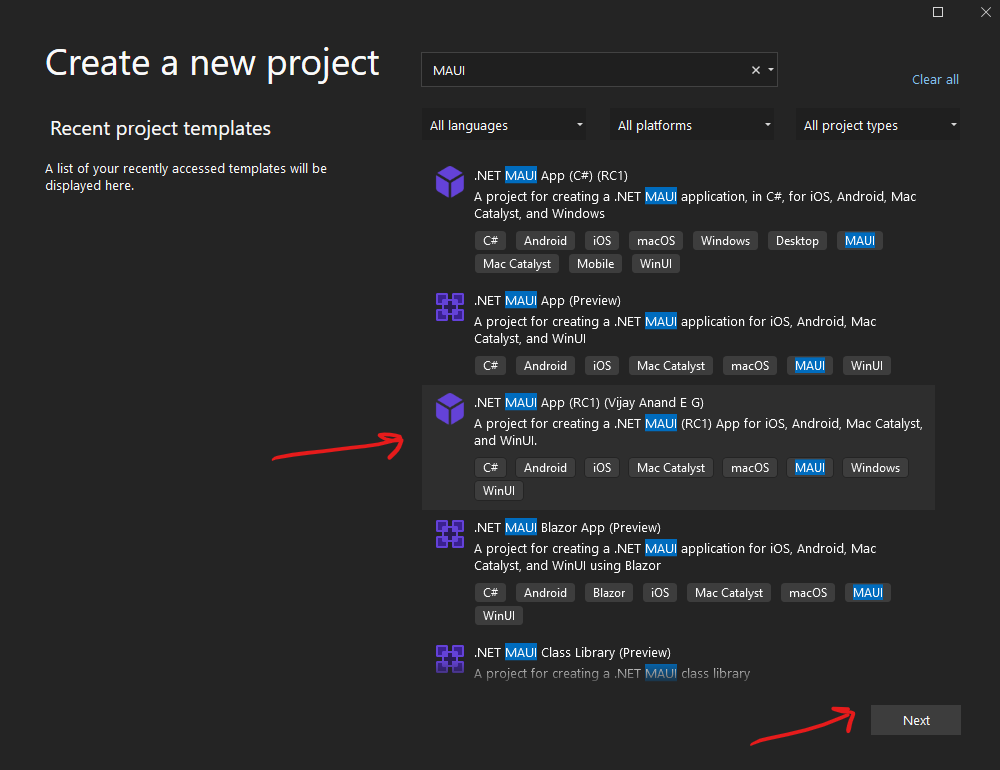
I selected the template I wanted, then I pressed the ‘Next’ button, to get started.
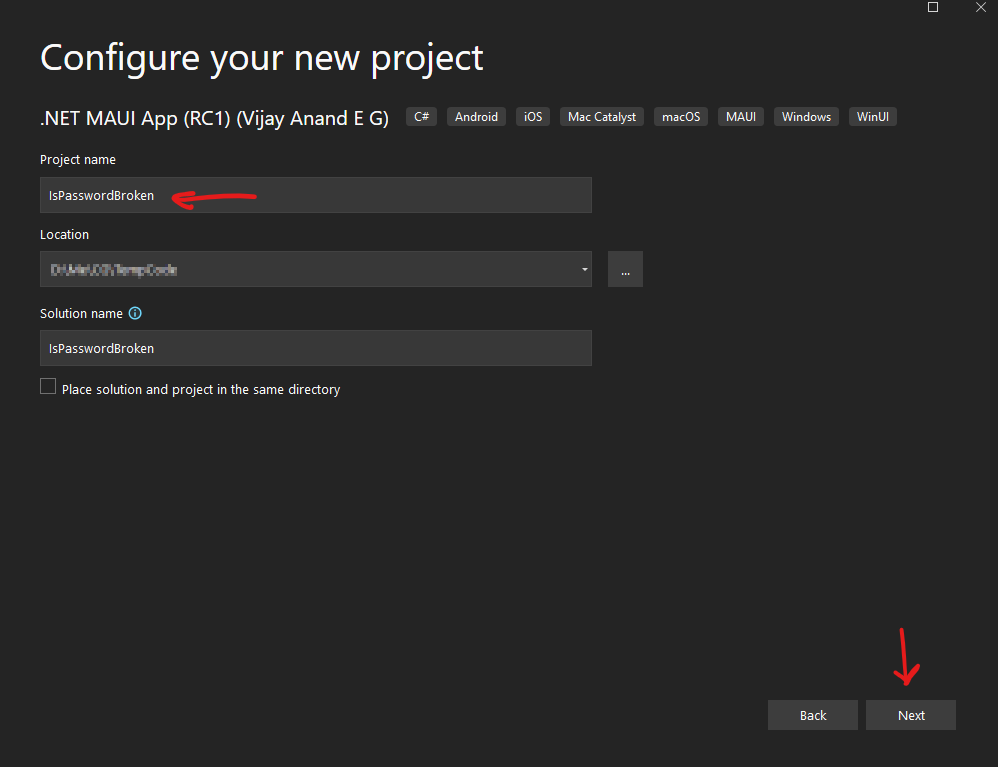
I gave the project a name, then I pressed the ‘Next’ button.
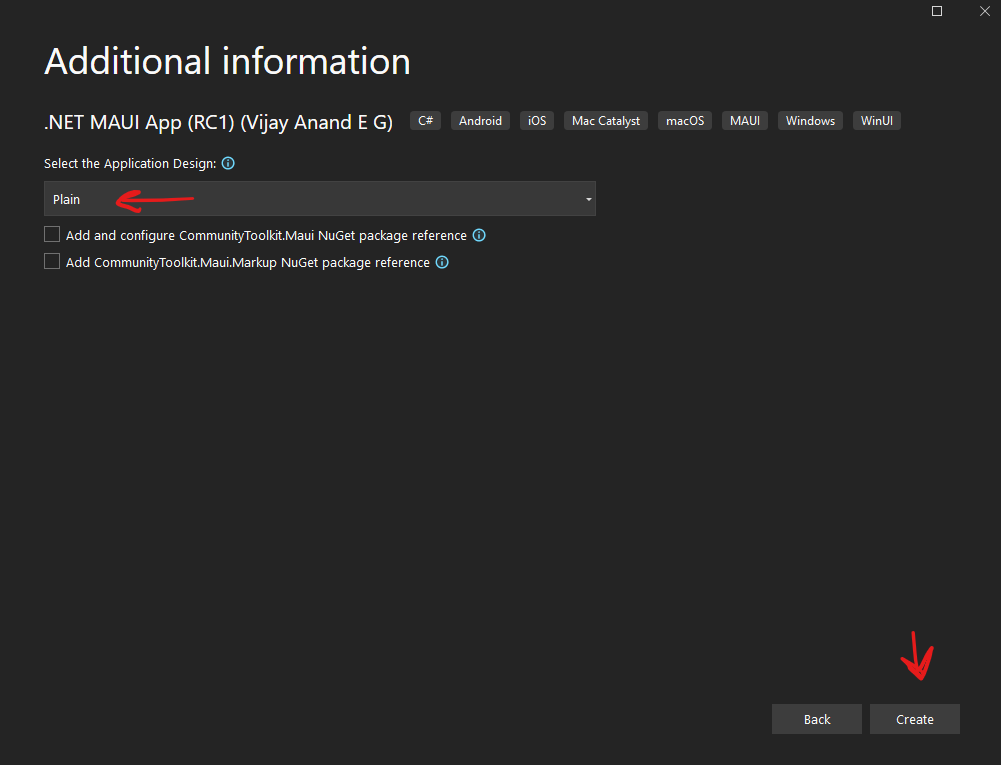
Then I chose a ‘plain’ project type, and pressed the ‘Create’ button.
That left me with this project structure:
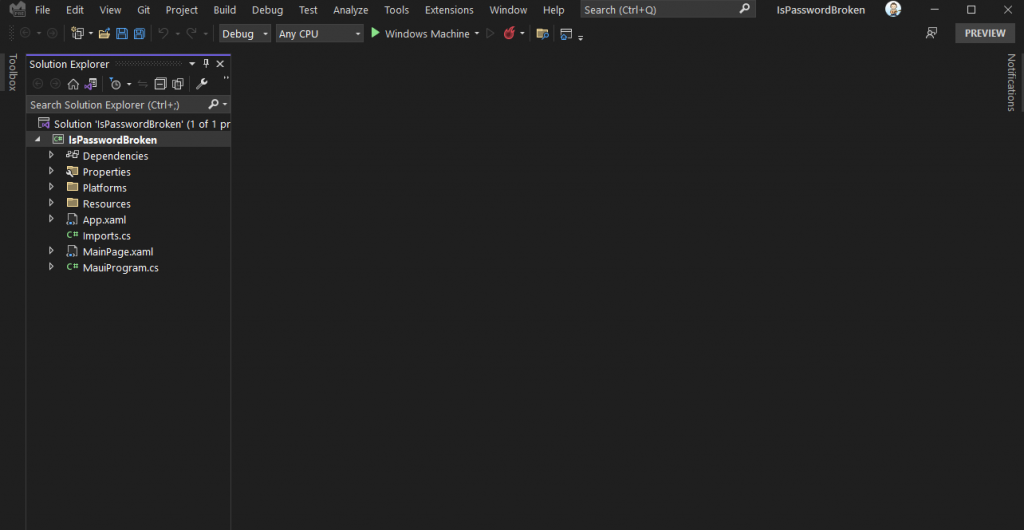
Then I opened the MainPage.xaml file and replaced the contents with this:
<?xml version="1.0" encoding="UTF-8" ?> <ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" x:Class="IsPasswordBroken.MainPage"> <ScrollView> <StackLayout Padding="30" Spacing="25"> <Entry IsPassword="True" Text="{Binding Test}" /> <Button x:Name="show" Text="Show Me!" Clicked="show_Clicked" /> </StackLayout> </ScrollView> </ContentPage>
So, I have a control with a single Entry
control, and a Button
. The Entry
control is bound to a property called Test
. The button is bound to a handler called show_Clicked
.
Next I edited the code-behind, for this page, making it look like this:
using System.ComponentModel; namespace IsPasswordBroken { public partial class MainPage : ContentPage, INotifyPropertyChanged { string _test = "this is a test"; public event PropertyChangedEventHandler? PropertyChanged; public string Test { get { return _test; } set { _test = value; PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(nameof(Test))); } } public MainPage() { BindingContext = this; InitializeComponent(); } private void show_Clicked(object sender, EventArgs e) { _ = DisplayAlert("Popup", $"The property value is: '{_test}'", "OK"); } } }
So, here I implemented the INotifyPropertyChanged
interface, with it’s PropertyChanged
event. Next I added a property named Test
. Next I set the BindingContext
, for the page, to the page, since we’re acting as our own view-model, for this simple demonstration. Finally, I implemented the show_Clicked
handler, so that it pops up the value of the Test
property, on demand.
When run on the Android emulator, the UI looks like this:
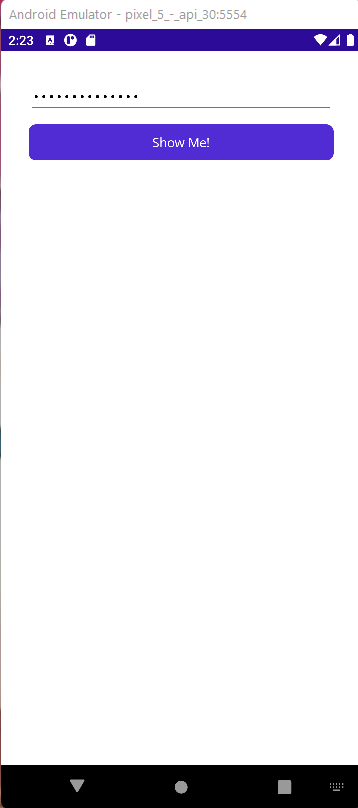
So, not bad! We see the Entry control, obviously bound to our Test property because we see a bunch of ‘*’ characters in the UI. We can verify that the underlying property contains some text by pressing the ‘Show Me!’ button. That looks like this:
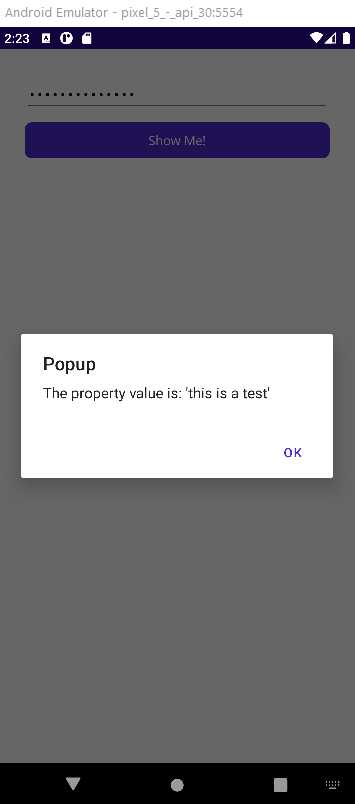
Terrific! So far, so good. Now let’s rebuild the application for Windows …
Here is what it looks like, as a Windows app:
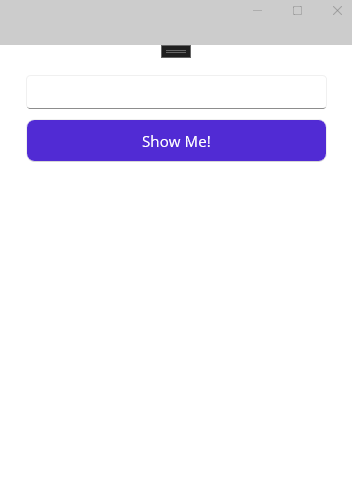
Hmm … Not exactly what we wanted. Maybe the binding failed? Let’s go check that …
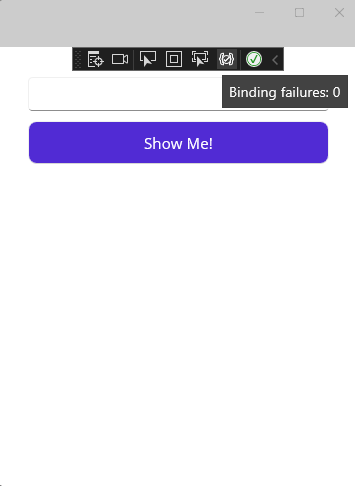
Nope, it must be binding alright. Let’s press the button to make sure the property contains some text …
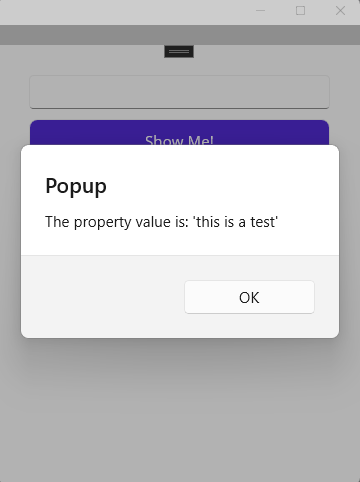
So, the binding is working, and the underlying property contains our text. What gives?? Let’s set the IsPassword
attribute to False, in the XAML:
<Entry IsPassword="False" Text="{Binding Test}" />
That looks like this:
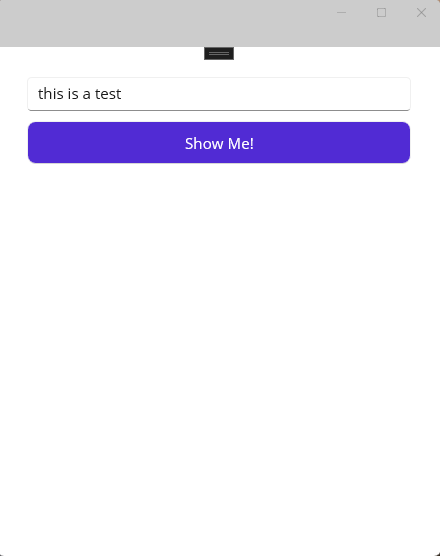
So, pretty safe to say, at this point, the .NET MAUI Entry
control has a bug that breaks the control whenever the IsPassword
attribute is set to True – At least, when running on Windows.
I was too lazy to try this same thing on iOS, since Visual Studio suddenly decided it can’t find my MAC. I’m too tired to delve into whatever that problem is …
Something else, I noticed, while writing all this out. When entering new text into the control, when the IsPassword
attribute is set to True, the text is mirrored, for some reason. Here is what is looks like when I typed ‘I changed this!’ in the field, then pressed the ‘Show Me!’ button:
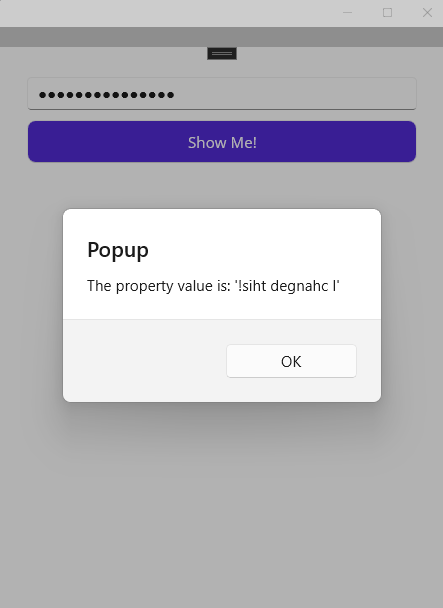
Nice, huh? Obviously, I could mirror the text again, if I wanted to work with this string. But, why should I have to do that? Not that it matters, since, with the broken binding in the Entry
control, I can’t ever use it with the IsPassword
attribute set to true anyway.
Don’t get me wrong. Overall, MAUI is awesome! But, little things like this make it difficult to put out a commercial quality application.
I hope Microsoft fixes these bugs, soon.
Photo by Mick Haupt on Unsplash